Learn the Random Gradient Generator in HTML, CSS and Javascript - Step by Step
Welcome to this relatively simple and fun project that will use HTML, CSS Javascript to create a Webpage that will randomly generate a gradient background
Click here to see what it will look like
Part 1 - Set up a basic HTML page
- First we need to start with some basic HTML - this is the structural language of webpages. No matter how complex web pages get, they rest on this foundation
- The code below will set up a very simple stucture which can apply to all web pages. We will use this to build up the content and styling of the page, not just for this project, but for pretty much all of them.
- To get started, copy this code into an editor and save as gradient.html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>My Gradient Page</title>
</head>
<body>
<h1>Welcome to my Gadient Generator</h1>
</body>
</html>
So what is actually going on?
It's pretty simple really, you surround the information you want with tags and every opening tag has a closing one
Part 2 - Let's start with a simple HTML setup for the Gradient Page
- To make the gradient generator, you need to add some paragraphs, a button and a hyperlink
- Copy this code into you page between the body tags (replacing your old H1 element)
<div class="container">
<div class="hex-colors">
<h1>Begin learning - Click the button below to randomise the hex colours</h1>
<h2>The hex code of the colours are: <span id="hex-code">#000000 , #000000</span></h2>
<p> </p>
<button onclick="changeColor()" class="btn btn-primary">Click Me</button>
<p> </p>
<p> </p>
<p> </p>
<p><a href="learngradient.html">Click here to learn</a></p>
</div>
</div>
So what is actually going on?
There are a couple of things we will ignore for now such as the #000000 hex numbers and the changecolor() reference
<Div> | <Span> | <P> | <A Href> | <Button> |
---|---|---|---|---|
A div is a section of a page that can be named and we can later give it some rules on how to bahave (size, colout, etc) | A span is just a section of the page we can give rules to (like a div but smaller) | A new paragraph so not everything is on the same line | a is the HTML for a link and href is the reference of where the link is to go - a hyperlink | A standard page button that can trigger an event - this will be used to trigger the gradient change |
Click here to see what it will look like
Part 3 - Let's add some basic bootstrap styling
- Add this line of code in between the HEAD tags:
<link rel="stylesheet" href= "https://stackpath.bootstrapcdn.com/bootstrap/4.5.2/css/bootstrap.min.css">
So what is actually going on?
Here we are just linking some styling rules to make make the font and button look slightly different. This comes from a bootstrap library which is a bunch of preset rules and stylings
Click here to see what it will look like
Part 4 - Let's add our own styling
- The following code needs to also go in between the head tags
- This language is called CSS (cascading style sheets)
<STYLE>
@import url('https://fonts.googleapis.com/css2?family=Comfortaa:wght@500&display=swap');
body {
font-family: 'Comfortaa', cursive;
}
a {
text-decoration: none;
}
a:hover {
text-decoration: underline;
font-weight: bold;
}
.container {
width: 95%;
margin: auto;
display: flex;
justify-content: center;
align-items: center;
height: 100vh;
}
.hex-colors {
width: 100%;
text-align: center;
}
.btn-primary {
display: block;
height: 50px;
width: 150px;
border-radius: 5%;
margin: 0 auto;
background-color: #333333;
border: none;
transition: all 1s ease;
transform: scale(1);
}
.btn-primary:hover {
background-color: #111111;
transform: scale(1.05) perspective(1px);
}
.hex-colors h1 {
text-transform: uppercase;
font-size: 2rem;
/*animation: colorchange 5s infinite alternate; */
}
.hex-colors h2 {
margin-top: 15%;
font-size: 2rem;
}
@keyframes colorchange {
0% {
color: indigo;
}
20% {
color: blue;
}
40% {
color: green;
}
60% {
color: yellow;
}
80% {
color: orange;
}
100% {
color: red;
}
}
@media (max-width: 480px) {
.hex-colors h1 {
font-size: 1.5rem;
}
.hex-colors h2 {
font-size: 1.5rem;
}
}
</STYLE>
So what is actually going on?
Let's break down some of the sections:
- >
- Body: This tells everything in the body how to behave. In this case it determines the font
- a: This tells hyperlinks how to act. In this case we want to get rid of the classic underline
- a:hover: This tells the hyperlink to to act when the mouse hovers over it (it makes it bold and underlined)
- .Container: The dot means class. So this tells the data within the container class how to behave. As we have set all content within this we can use this to set the width and alignment of the content
- btn-primary: This is the button for changing the gradient. I have set the colour as well as some other effects
- @Media: This alters the font size if a mobile pohone screen is detected
Click here to see what it will look like
Now the page looks great apart from the fact that it doesn't actually do anything. Now we will give it some script but first you need to know a bit about hex colours:
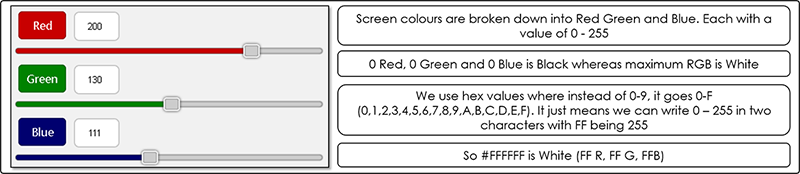
Part 5 - Let's give it some functionality with some javascript
- The following javascript can be inserted just above the </BODY> tag
- Finally we should have the ablity for the user to control the page
<script>
function changeColor() {
var hex_numbers = ["0", "1", "2", "3", "4", "5", "6", "7", "8", "9", "A", "B", "C", "D", "E", "F"];
var hexcode = '';
var hexcode2 = '';
for (var i = 0; i < 6; i++) {
var random_index = Math.floor(Math.random() * hex_numbers.length);
hexcode += hex_numbers[random_index];
var random_index2 = Math.floor(Math.random() * hex_numbers.length);
hexcode2 += hex_numbers[random_index2];
var startval1 = "#" + hexcode;
var startval2 = "#" + hexcode2;
}
document.getElementById("hex-code").innerHTML = '#' + hexcode + ', #' + hexcode2;
document.body.style.backgroundImage = "linear-gradient(to right, " + startval1 + ", " + startval2 + ")";
}
</script>
So what is actually going on?
Let's break down some of the sections:
var hex_numbers = ["0", "1", "2", "3", "4", "5", "6", "7", "8", "9", "A", "B", "C", "D", "E", "F"];
This creates a list of all possible hex digits that we can rondomly reference
function changecolor() | var hexcode = '' | for (var i = 0; i < 6; i++) |
---|---|---|
A function is a section of code that does a job. We only have one function that is called by the botton in the HTML | This is a variable that holds a 6 digit hexcode (we need two of these) | This is a loop. it loops 6 times and we use the counter 'i' to reference a hex number in the 6 digit sequaence |
var random_index = Math.floor (Math.random() * hex_numbers.length); |
var startval1 = "#" + hexcode; | document.body.style .backgroundImage = "linear-gradient(to right, " + startval1 + ", " + startval2 + ")"; |
---|---|---|
This calls in the random number generator to generate a number to access the list of hex numbers | now we assign the hexcode with the # to create a code to reference | Now we can use the two hex numbers to create the random gradient and aply it to the body of the document |
Click here to see what it will look like